ASP.NET API Backend: Secure File Uploads with Mime-Detective
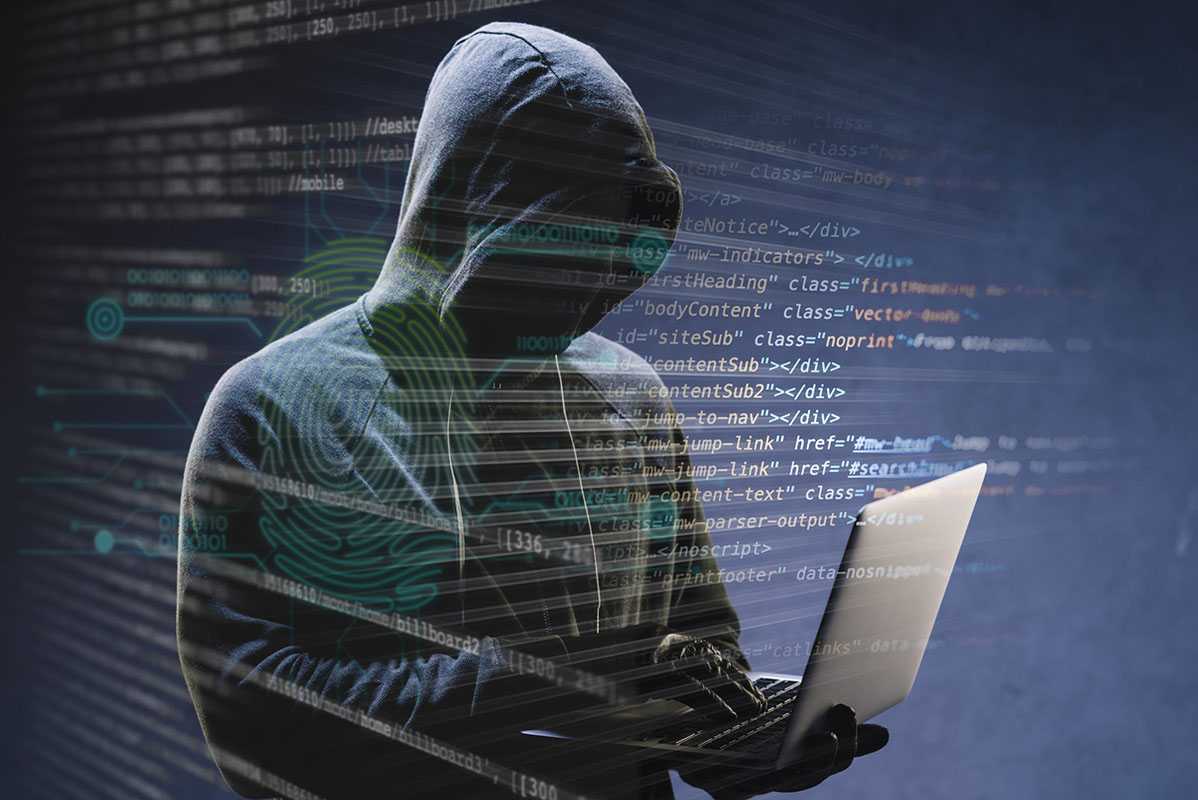
In today’s web applications, handling user-uploaded files is a common requirement. While allowing file uploads opens up functionalities, it also introduces security concerns. Malicious users might try to upload files with inappropriate content or exploit vulnerabilities in your application. Implementing robust file validation is crucial to safeguard your ASP.NET API back-end.
This article explores how to validate file uploads based on their content (MIME type) using Mime-Detective, a popular .NET library. By relying on MIME types instead of solely on file extensions, you can ensure a more secure and reliable file upload process.
Why Content-Based Validation with Mime-Detective?
Traditionally, file extensions (e.g., .docx
, .pdf
) have been used to validate uploaded files. However, this approach can be easily bypassed. Users can rename files with malicious intent, making them appear as legitimate types.
Mime-Detective offers a more secure solution. It examines the actual content of the uploaded file, typically the first few bytes, to identify the true MIME type. This signature-based approach helps prevent unauthorised file types from entering your system.
Steps for Secure File Upload Validation
- Install Mime-Detective: Use NuGet Package Manager to add the “Mime-Detective” package to your ASP.NET API project.
- Access Uploaded File: Within your API controller method that handles file uploads, you’ll typically have access to the uploaded file through an
IFormFile
object. This object provides properties likeContentLength
andMemoryStream
to access the file data. - Read File Bytes: Read a small chunk of bytes (usually the first few hundred) from the uploaded file’s
MemoryStream
into a byte array. - Use Mime-Detective:
- Instantiate a
ContentInspector
object using Mime-Detective's default definitions. - Call the
Inspect
method on theContentInspector
instance, passing the byte array containing the file data. This method attempts to identify the MIME type based on the file signature.
5. Validate MIME Type:
- Check the results returned by
Inspector
. If a MIME type is identified: - Compare it against a list of allowed MIME types for your application.
- If there’s a match, proceed with processing the uploaded file.
- If no MIME type is found or it’s not allowed, return an error response to the client indicating the file type is invalid.
Code Implementation Example (ASP.NET Core):
public async Task<IActionResult> FileUpload([FromForm] FileUploadModel model)
{
try
{
if (model?.File == null || model.File.Length <= 0)
{
return BadRequest("No file was uploaded.");
}
// Read the file content
using (var ms = new MemoryStream())
{
await model.File.CopyToAsync(ms);
var fileBytes = ms.ToArray();
var Inspector = new ContentInspectorBuilder()
{
Definitions = MimeDetective.Definitions.Default.All()
}.Build();
var result = Inspector.Inspect(fileBytes);
string fileType;
if (result.Length > 0)
{
fileType = result.FirstOrDefault().Definition.File.MimeType;
}
if (true) // Replace with your logic to check allowed types
{
// Process and save the uploaded file
// ...
return Ok("File uploaded successfully!");
}
else
{
return BadRequest("Invalid file format. Only [list of allowed types] allowed!");
}
}
}
catch (Exception e)
{
return BadRequest(e.Message);
}
Additional Considerations:
- Error Handling: Implement proper error handling and logging for exceptions during file upload or Mime-Detective processing.
- Buffer Size: Consider adjusting the buffer size (
bytesToRead
) depending on the complexity of file signatures you want to detect. - Custom MIME Types: You can extend Mime-Detective’s definitions to handle custom MIME types specific to your application.
By following these steps and incorporating Mime-Detective, you can significantly enhance the security and reliability of your ASP.NET API’s file upload functionality. Remember to stay updated with the latest security best practices to maintain a robust and secure back-end for your web application.